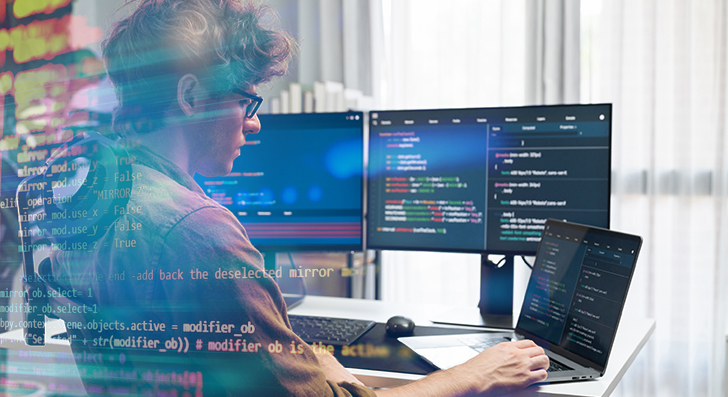
Scalability indicates your software can take care of progress—a lot more users, extra facts, and a lot more targeted traffic—without having breaking. As being a developer, setting up with scalability in your mind saves time and stress afterwards. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Numerous purposes fail if they develop rapid since the first style can’t cope with the extra load. To be a developer, you should Imagine early about how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Steer clear of monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial components. Just about every module or service can scale on its own with no influencing the whole program.
Also, contemplate your databases from day a person. Will it require to manage 1,000,000 buyers or perhaps 100? Pick the right sort—relational or NoSQL—based on how your facts will grow. Program for sharding, indexing, and backups early, Even though you don’t need them but.
One more significant point is to prevent hardcoding assumptions. Don’t create code that only performs underneath current circumstances. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design patterns that assistance scaling, like concept queues or function-pushed methods. These support your application take care of more requests without the need of obtaining overloaded.
Whenever you Develop with scalability in mind, you are not just making ready for fulfillment—you are lowering potential head aches. A nicely-planned procedure is less complicated to take care of, adapt, and improve. It’s superior to get ready early than to rebuild afterwards.
Use the appropriate Database
Selecting the right databases is often a critical Section of developing scalable applications. Not all databases are constructed exactly the same, and utilizing the Erroneous one can slow you down or maybe lead to failures as your app grows.
Get started by knowledge your knowledge. Could it be very structured, like rows inside a desk? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. These are generally robust with relationships, transactions, and consistency. Additionally they help scaling methods like browse replicas, indexing, and partitioning to manage much more targeted visitors and knowledge.
In case your facts is much more flexible—like person activity logs, item catalogs, or files—take into account a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at managing huge volumes of unstructured or semi-structured details and may scale horizontally a lot more easily.
Also, take into consideration your go through and generate patterns. Will you be performing numerous reads with fewer writes? Use caching and browse replicas. Are you managing a hefty publish load? Take a look at databases that will take care of superior compose throughput, or maybe event-primarily based knowledge storage techniques like Apache Kafka (for momentary data streams).
It’s also intelligent to Consider in advance. You might not need Superior scaling capabilities now, but deciding on a databases that supports them indicates you received’t need to switch later on.
Use indexing to speed up queries. Keep away from avoidable joins. Normalize or denormalize your data based on your accessibility designs. And normally observe database effectiveness when you mature.
In short, the proper database is determined by your app’s framework, pace demands, And exactly how you expect it to grow. Consider time to pick properly—it’ll conserve plenty of problems later on.
Optimize Code and Queries
Quick code is vital to scalability. As your application grows, every little delay provides up. Improperly penned code or unoptimized queries can decelerate general performance and overload your procedure. That’s why it’s imperative that you Make successful logic from the start.
Start out by composing clear, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t select the most complicated solution if a straightforward a single will work. Keep your capabilities limited, targeted, and straightforward to test. Use profiling tools to uncover bottlenecks—spots exactly where your code takes far too extended to run or takes advantage of excessive memory.
Up coming, take a look at your databases queries. These frequently gradual issues down much more than the code by itself. Make sure Just about every query only asks for the info you really have to have. Keep away from Choose *, which fetches anything, and rather pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Specifically across massive tables.
If you observe the same knowledge remaining requested time and again, use caching. Store the final results quickly making use of applications like Redis or Memcached which means you don’t need to repeat high-priced functions.
Also, batch your databases operations if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application more effective.
Remember to take a look at with huge datasets. Code and queries that get the job done great with 100 information may possibly crash every time they have to handle 1 million.
In brief, scalable apps are quickly applications. Maintain your code limited, your queries lean, and use caching when required. These measures support your software keep clean and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it has to handle more customers and even more targeted traffic. If everything goes through 1 server, it'll speedily become a bottleneck. That’s the place load balancing and caching are available in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic across various servers. Instead of a person server accomplishing many of the get the job done, the load balancer routes people to diverse servers depending on availability. This means no one server receives overloaded. If one particular server goes check here down, the load balancer can deliver traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts temporarily so it can be reused promptly. When consumers request the exact same data once more—like an item website page or maybe a profile—you don’t must fetch it from the databases each and every time. You can provide it in the cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) outlets info in memory for fast entry.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the consumer.
Caching reduces database load, increases speed, and will make your app additional effective.
Use caching for things which don’t alter usually. And normally ensure your cache is current when info does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with more end users, continue to be quick, and Get well from problems. If you plan to increase, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you may need instruments that permit your app expand simply. That’s where by cloud platforms and containers come in. They give you adaptability, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t should invest in components or guess upcoming capacity. When visitors raises, you'll be able to increase more resources with just a few clicks or immediately utilizing automobile-scaling. When visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to creating your app rather than managing infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most well-liked Instrument for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person aspect of one's application crashes, it restarts it routinely.
Containers also allow it to be easy to individual elements of your application into providers. You'll be able to update or scale parts independently, and that is great for performance and dependability.
In short, working with cloud and container resources usually means it is possible to scale quick, deploy quickly, and Recuperate promptly when issues materialize. If you'd like your application to develop devoid of limits, start off making use of these applications early. They conserve time, cut down danger, and make it easier to stay focused on making, not fixing.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is carrying out, location issues early, and make far better selections as your application grows. It’s a key Portion of making scalable units.
Begin by tracking standard metrics like CPU utilization, memory, disk House, and reaction time. These tell you how your servers and solutions are carrying out. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your application too. Keep watch over just how long it requires for end users to load web pages, how frequently glitches transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital complications. For example, if your reaction time goes higher than a Restrict or maybe a provider goes down, you should get notified immediately. This helps you take care of challenges rapid, generally ahead of end users even recognize.
Monitoring is usually handy after you make variations. For those who deploy a different characteristic and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic injury.
As your app grows, targeted visitors and details raise. Without having checking, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t only for large corporations. Even little applications require a robust foundation. By planning cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Establish intelligent.